Python Decorators Explained for Beginners
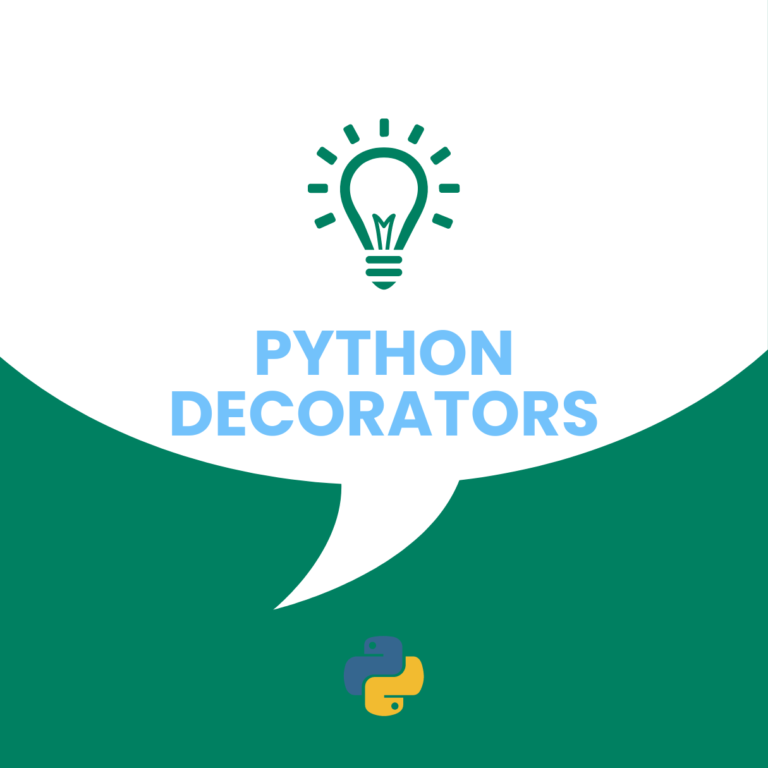
Python decorators are considered one of the very advanced and very useful concepts. In this article, I will explain Python decorators with examples. Going through this article, you will learn to implement Python decorators and how they can be very useful in your project.
What is Python Decorators?
Python decorators is a technique for changing the behavior of an existing function without changing the actual code inside the function.
Even though we are changing the behavior of the function, there should not have any change in the function definition and function call.
It means…
- The code inside the original function should not be changed.
- There should not have any change in the function call.
Python has a special mechanism called Python decorators to change the behavior of the existing function.
Let’s go with an example.
def myFunc():
print("Hamropedia is an amazing Website.")
myFunc()
Output:
Hamropedia is an amazing Website.
This is the basic Python program to implement a Python function. Now consider a function that you want to wrap inside another function which is called a decorator.
We know, everything in Python is an object including the Python function. It means, we can pass the function object as an argument to another function. By default, the function name works as a function object.
We can write one Python function inside another function.
Decorator Code 1st
def myWrapper(func):
def myInnerFunc():
print("Read an amazing article.")
func()
return myInnerFunc
def myFunc():
print("Hamropedia is an amazing Website.")
myFunc=myWrapper(myFunc)
myFunc()
Output:
Read an amazing article.
Hamropedia is an amazing Website.
Here we are passing the function (myFunc) as a parameter to the wrapper function (myWrapper).
This is how it works.
- The actual function is passed as a parameter to the wrapper function.
- myWrapper has an inner function that calls the actual function (myFunc).
- Inside the Inner function, you can write any additional operation to perform along with calling the original function (myFunc).
- myWrapper returns the inner function object.
Note: When you write a function name without brackets, it acts as a function object and it does not call a function. The function gets called only when there is a function name (object) followed by brackets “()”.
If you compare the above two programs, you have changed the behavior of the original function. You can see the difference in the output.
We have fulfilled the first criterion of not changing code inside the function. But the function call has changed
from
myFunc()
to
myFunc=myWrapper(myFunc)
myFunc()
This does not fulfill our second criterion. Here is the use of Python decorators. But don’t worry we can replace our
myFunc=myWrapper(myFunc)
to
@myWrapper
But this @myWrapper should always be written on the top of the original function, in our case, it is the myFunc function.
Decorator Code 2nd
def myWrapper(func):
def myInnerFunc():
print("Read an amazing article.")
func()
return myInnerFunc
@myWrapper
def myFunc():
print("Hamropedia is an amazing Website.")
myFunc()
Output:
Read an amazing article.
Hamropedia is an amazing Website.
But the Decorator Code 1st and Decorator Code 2nd give the same output in both cases.
When to use Python Decorators?
Suppose you are working on a project. You are asked to make changes in a certain complex function.
If you are making changes in already tested and robust functions, you can not deny the possibility of breaking functionality.
A better way is to use Python decorators.
- You don’t need to make changes to the already-tested function.
- You don’t need to make any changes to the function call. This will be very useful if there are multiple function calls in your project source code.
This is all about Python decorators explained with examples.
Categories
Popular Posts
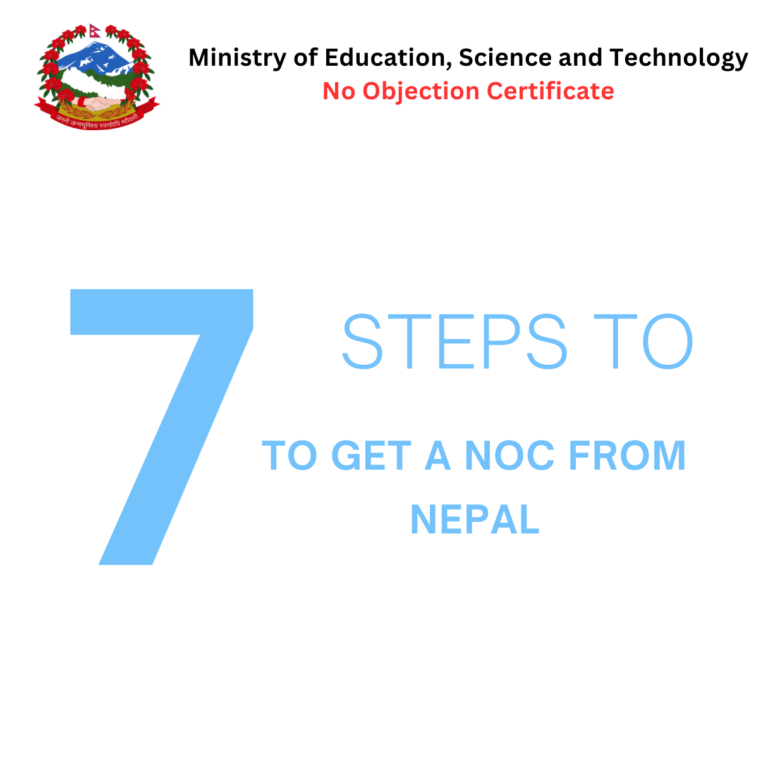
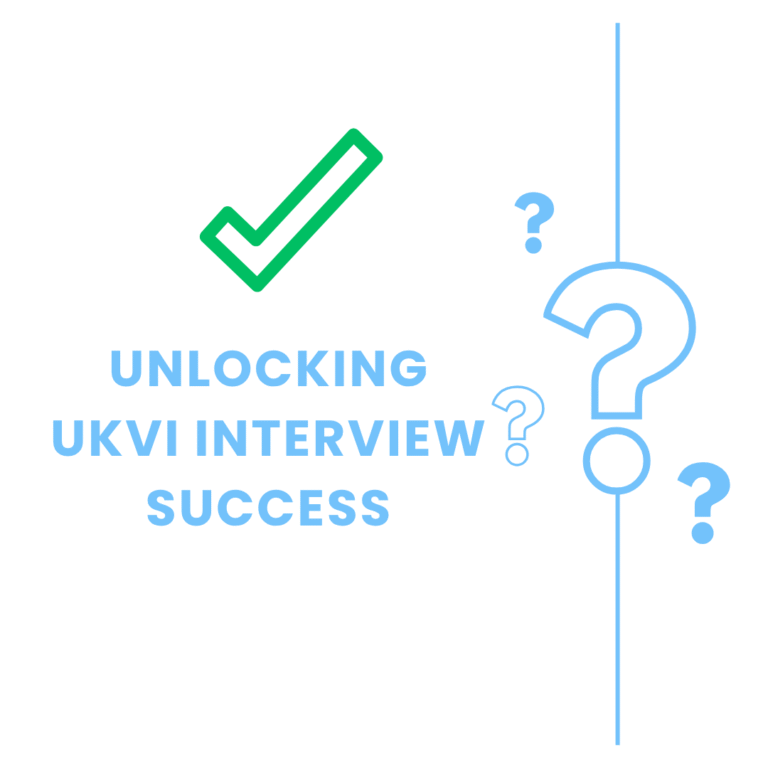
Top UKVI Interview Question Examples and Answers
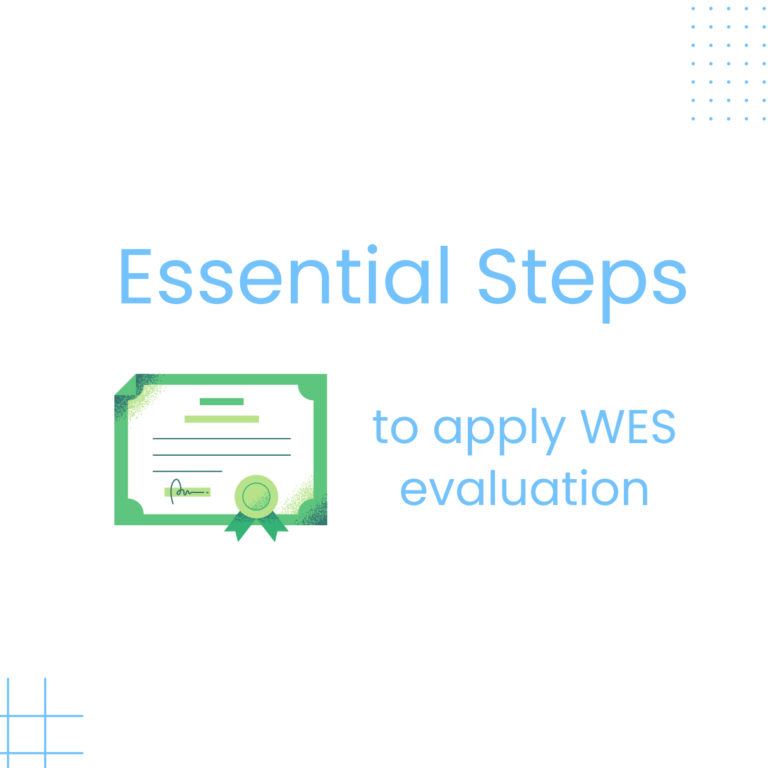
How to Apply for WES Evaluation in 2025?
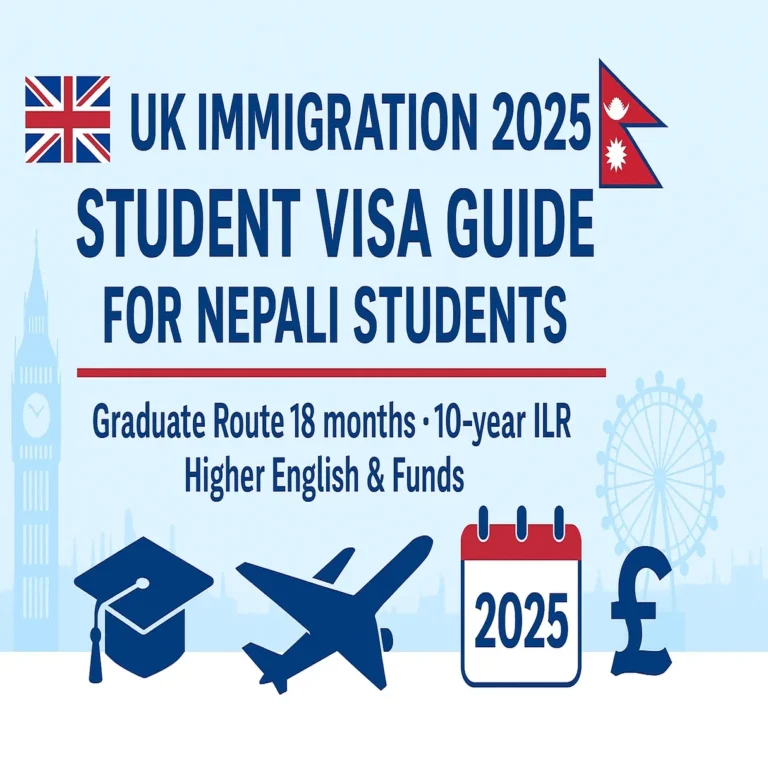